A Primer in Computer Programming
Many introductory courses in computer programming starts with Turtle Graphics, especially when based on the Logo programming language. The methodical concept we present here is almost the same and we apply it in 5 steps. Using Java has the clear advantage that you are not limited to turtle graphics, but you have the power of a full-featured and widely accepted higher programming language that you can use when you get more skilled. Your learning process is open-ended and developing professional Android apps is an option for you without passing to another language.
Step 1: Programming is commanding the turtle: "do this, then do that, then..."
(Notion to learn: "Sequence" of statements)
package turtle.tut04;
import turtle.*;
public class Tut04 extends Playground
{
public void main()
{
st();
fd(100);
rt(90);
fd(100);
rt(90);
fd(100);
rt(90);
fd(100);
rt(90);
}
}
|
|
Open source in Online-Compiler. (You need the latest version of JRE.)
Create QR code to download Android app to your smartphone.
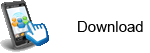 |
source (Tut04.zip). |
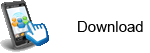 |
Android app for installation on a smartphone or emulator. |
Step 2: Programming is repeating blocks: "Repeat a certain number of times the following command"
(Notion to learn: "Repeat" program structure)
import turtle.*;
package turtle.tut05;
import turtle.*;
public class Tut05 extends Playground
{
public void main()
{
st();
for (int i = 0; i < 4; i++)
{
fd(100);
rt(90);
}
}
}
|
|
Open source in Online-Compiler. (You need the latest version of JRE.)
Create QR code to download Android app to your smartphone.
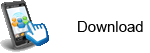 |
source (Tut05.zip). |
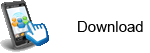 |
Android app for installation on a smartphone or emulator. |
Step 3: Programming is creating named blocks: "These statements belongs to a unity with name.."
(Notions to learn: Method declaration, method call)
package turtle.tut06;
import turtle.*;
public class Tut06 extends Playground
{
public void main()
{
st();
setSpeed(MAXSPEED);
for (int i = 0; i < 60; i++)
{
square();
rt(6);
}
}
void square()
{
for (int i = 0; i < 4; i++)
{
fd(100);
rt(90);
}
}
}
|
|
Open source in Online-Compiler. (You need the latest version of JRE.)
Create QR code to download Android app to your smartphone.
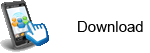 |
source (Tut06.zip). |
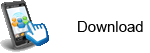 |
Android app for installation on a smartphone or emulator. |
Step 4: Passing values to a named block: "The method gets the value of parameter (argument) 'side'"
(Notions to learn: Method parameters (arguments), formal parameter, actual parameter)
package turtle.tut07;
import turtle.*;
public class Tut07 extends Playground
{
public void main()
{
for (int i = 0; i < 60; i++)
{
square(2 * i);
rt(6);
}
}
void square(double side)
{
for (int i = 0; i < 4; i++)
{
fd(side);
rt(90);
}
}
}
|
|
Open source in Online-Compiler. (You need the latest version of JRE.)
Create QR code to download Android app to your smartphone.
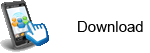 |
source (Tut07.zip). |
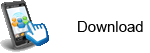 |
Android app for installation on a smartphone or emulator. |
Step 5: A named block (method) may call itself, like in the joke "To understand recursion, you must understand recursion"
(Notions to learn: Recursion, recursion anchor)
package turtle.tut08;
import turtle.*;
public class Tut08 extends Playground
{
public void main()
{
st();
setSpeed(MAXSPEED);
spiral(10);
}
void spiral(int s)
{
if (s > 200)
return;
fd(s);
rt(70);
spiral(s + 2);
}
}
|
|
Open source in Online-Compiler. (You need the latest version of JRE.)
Create QR code to download Android app to your smartphone.
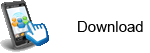 |
source (Tut08.zip). |
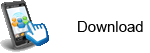 |
Android app for installation on a smartphone or emulator. |
Step 6: A turtle is an object and has its specific properties and abilities "'new' creates a new object instance"
(Notion to learn: Object oriented programming, OOP)
package turtle.tut09;
import turtle.*;
public class Tut09 extends Playground
{
Turtle joe = new Turtle(RED);
Turtle laura = new Turtle(GREEN);
public void main()
{
joe.setPenColor(RED);
joe.setPenWidth(10);
laura.setPenColor(GREEN);
for (int i = 0; i < 9; i++)
{
joe.forward(150).lt(160);
laura.forward(150).rt(160);
}
}
}
|
|
Open source in Online-Compiler. (You need the latest version of JRE.)
Create QR code to download Android app to your smartphone.
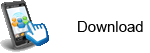 |
source (Tut09.zip). |
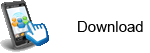 |
Android app for installation on a smartphone or emulator. |
Step 6: Objects can be classified in class hierarchies: "A PainterTurtle is a Turtle that has all abilities of a turtle, but additionally knows how to paint a flower"
(Notions to learn: Object identify, inheritance, multi-threading)
package turtle.tut10;
import turtle.*;
public class Tut10 extends Playground
{
public void main()
{
for (int i = 0; i < 30; i++)
new PainterTurtle();
}
class PainterTurtle extends Turtle
implements Runnable
{
PainterTurtle()
{
new Thread(this).start();
setHeading(360 * Math.random());
}
public void run()
{
fd(50 + 100 * Math.random());
lt(45);
for (int i = 0; i < 10; i++)
{
fd(50);
bk(50);
rt(20 * Math.random());
}
}
}
}
|
|
Open source in Online-Compiler. (You need the latest version of JRE.)
Create QR code to download Android app to your smartphone.
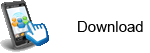 |
source (Tut10.zip). |
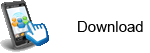 |
Android app for installation on a smartphone or emulator. |
(Continued in Tutorial 3)
|