The source code of all examples is included in the JGameGrid distribution.
How To Build Full-Fledged Professional Game Applications
We are going to present design hints how to build a professional game application using the JGameGrid framework. As usual we explain by an example that is generally enough to include elements of a widespread class of 2D games. The game design consists of a game area with a graphics background, called game pane, where the game actors interacts. Input-output controls for the user interaction are shown in a separate area, called navigation pane.
Because we also want to have animated graphics in the navigation pane, it is a good idea to use a GameGrid instance for the game pane as well for the navigation pane. Because a GameGrid is a java.awt.Canvas it can be embedded as component into the content pane of a JFrame (as already shown in Ex02). In a more complex game design, additional components (e.g. additional GameGrids, JPanels with standard Swing controls, GPanes from the ch.aplu.util package, etc.) could be shown in the same JFrame.
As an example we use the well-known game "Snakes and Ladder". For the game board design and the game rules, consult the Wiki site, which is simple enough not to blow-up the code tremendously. The implementation presented here a only for demonstration purposes. It is up to you to complete the implementation and build a a real game for two or more players on the same computer, for one player against a computer player or even for several players connected via TCP or Bluetooth.
To make you greedy we show the result and let you play right now in order you have an overview of the basic behavior we want to implement.
Execute the program locally using WebStart.
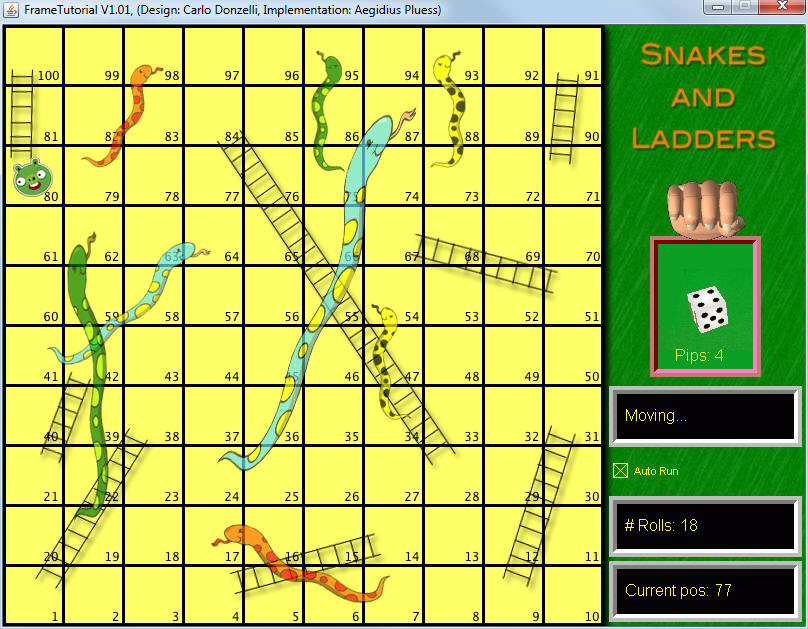
As you see, the game clearly has a professional look (thanks to Carlo Donzelli for the graphical design). Even the rolling die is animated when the hand is clicked. OOP helps you to keep the code manageable. We use the following class design with the general concept to delegate specific tasks to specific classes and use few interconnections (for calling methods and passing information) between the classes.
FrameTutorial: |
Application class derived from JFrame. Creates the GamePane and NavigationPane instances |
GamePane: |
Class derived from GameGrid. A grid of 10x10 cells (60 pixel size) is used. The visible grid lines, snakes and ladders are part of the background image. Has-a list of connections that represents the snakes and ladders logically |
NavigationPane: |
Class derived from GameGrid. Defines the layout of the user input/output controls. Implements a GGButtonListener to get buttonClicked notifications when the hand button is clicked. This callback launches a new Die instance that shows a rolling die. The end of the die rolling simulation is reported back by calling startMoving() where the go() method of the Puppet class starts the puppet movement (we don't enable the Die class to command the puppet directly) |
Puppet: |
Class derived from Actor. Represents the moving object. Moves the object to cells with increasing cell indices corresponding to the current number of pips. Checks if the movement end on a ladder bottom or snake head. If this is the case, moves the object to the connection ending cell. Keep in mind that in JGameGrid the animation dynamics is automatically generated by the running simulation thread that calls act() periodically. Depending on the current actor state, act() must perform a specific code sequence and return. We use a Connection reference currentCon to inform act() whether it should move the actor in the grid (currentCon =0 null) or on the connection (currentCon != null). Never use a loop in act() to move an actor! |
Die: |
Class derived from Actor. Simulates the rolling die by 7 sprite images (spriteId = 0..6) of the same actor. Each of the 6 pips results is generated by a different Die instance. Once the die is generated, it is cycling automatically through its sprite images in the act() method (what again shows a fundamental feature of the JGameGrid design concept) |
Connection: |
Class that represents the directed link between two cells. The puppets move directly from the starting to the ending cell. This class is abstract to prevent instance creation |
Snake: |
Specialized class derived from connection that represents snakes |
Ladder: |
Specialized class derived from connection that represents ladders |
For demonstration purposes we include an Auto Run feature that generates the hand clicks automatically.
More than just a game
"Snakes and Ladder" is not only for fun, you can learn a lot about mathematical statistics. The game is an example of a "Markow Chain" with states 0 to 100, corresponding to the cell numbers 0 to 100 (state 0 is the invisible starting cell). The next state depends on the outcome of the rolling die, but does not depend on what happened before. Simple and more complicated questions may be asked for our particular game layout concerning the statistical behavior when the game is played many times (theoretically an infinite number of times), like
- What is the minimum number of rolls to reach the end position?
- What is the frequency distribution of the number or rolls?
- What is the number of rolls at the maximum of this distribution (the most likely number of rolls)?
- What is the probability to reach the end position with less or equal than a given number n of rolls?
- Is it possible to get blocked in an infinite loop and never reach the end position?
Without going into theoretical details, these question can be answered approximately by performing the game simulation a great number of times. Here the computer power comes in. We replace the human player by a computer player implemented as a Java thread that plays automatically as long as we want. Because there is no need of user input, we just display the frequency distribution in the right pane. Using the JGameGrid's GGPanel class, a pretty graphics is created effortlessly.
Execute the program locally using WebStart.
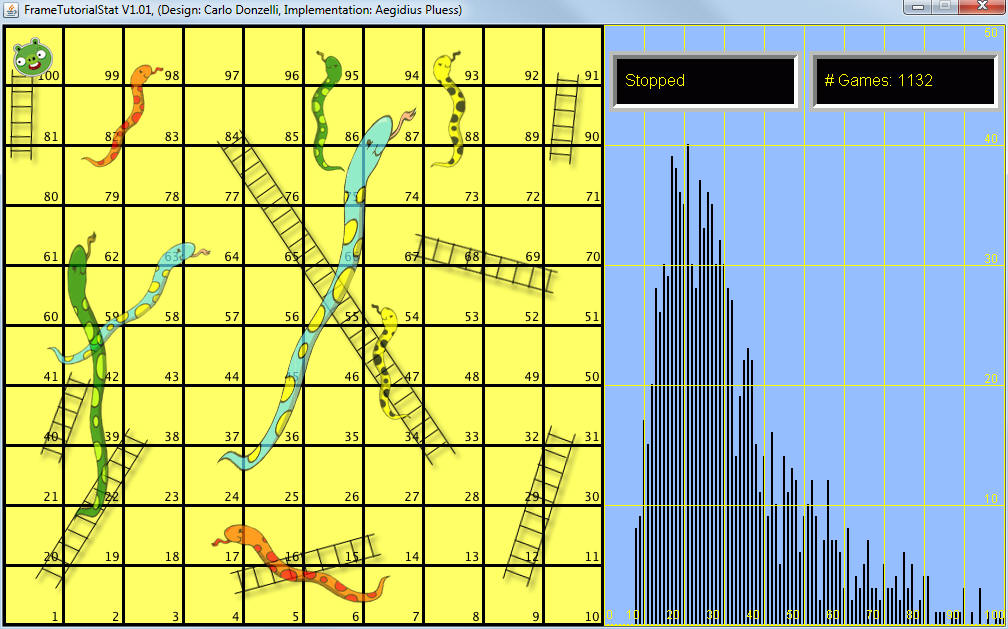
Try to give an answer to the five questions now!
|