|
The source code of all examples is included in the JGameGrid distribution.
Ex10: Paint and Act Order
In some applications it may be important which act() is called first, second, etc. We call this the "act order". By default this order depends which instance is added first, second, etc. to the GameGrid, thus by the calling sequence of addActor(). The default ordering is perforemd by classes: instances of classes added last will act first. Within a class, instances added last will act first. This gives some predominance of actors added at a later time over actors added before. This default act order may be changed at runtime by the setActOrder() method. In the following example we use the Bear class of Ex04 and a copy Dog of this class with the only difference that the icon is a dog instead of a bear. If you comment out the line with setActOrder() and run the program by pressing Run or better be pressing Step you see that the dog survives. If you change the order of the two addActor() lines or you do not comment out setActOrder() the bear survives. That's the way of life: who acts first will have a better chance to survive.
import ch.aplu.jgamegrid.*;
public class Ex10 extends GameGrid
{
public Ex10()
{
super(10, 10, 60, java.awt.Color.red);
addActor(new Bear(Dog.class), new Location(0, 5));
addActor(new Dog(Bear.class), new Location(9, 5), 180);
// setActOrder(Bear.class);
show();
}
public static void main(String[] args)
{
new Ex10();
}
} |
Execute the program locally using WebStart.
Execute the program with setActOrder() not commented out
Analog to the act order there is also an order of repainting the actor sprite images, called "paint order". This order determines which image is drawn "on top" of others. The default paint order is the same as with act order, actors added last will be drawn on top of actors added before. The default order may be changed with setPaintOrder().
In the following example a rotating earth is shown using 18 different sprite images earth_0.gif..earth_17.gif. In the class Earth the method act() cycles through these images by calling showNextSprite().
import ch.aplu.jgamegrid.*;
public class Earth extends Actor
{
private static final int nbSprites = 18;
public Earth()
{
super("sprites/earth.gif", nbSprites);
setSlowDown(6);
}
public void act()
{
showNextSprite();
}
}
|
act() in the class Rocket moves a rocket horizontally back and forth. At each turn, the paint order is flipped, thus the rocket will pass in front or behind the earth.
import ch.aplu.jgamegrid.*;
public class Rocket extends Actor
{
public Rocket()
{
super("sprites/rocket.gif");
}
public void act()
{
move();
if (getX() < -100 || getX() > 700)
{
turn(180);
if (isHorzMirror())
{
setHorzMirror(false);
gameGrid.setPaintOrder(Rocket.class); // Rocket in foreground
}
else
{
setHorzMirror(true);
gameGrid.setPaintOrder(Earth.class); // Earth in foreground
}
}
}
} |
The application class adds the rocket after the earth. Therefore as default the rocket will be drawn in front of the earth.
import ch.aplu.jgamegrid.*;
public class Ex10a extends GameGrid
{
public Ex10a()
{
super(600, 200, 1, null, false);
setSimulationPeriod(50);
Earth earth = new Earth();
addActor(earth, new Location(300, 100));
// Rocket added last->default paint order: in front of earth
Rocket rocket = new Rocket();
addActor(rocket, new Location(200, 100));
show();
doRun();
}
public static void main(String[] args)
{
new Ex10a();
}
} |
Execute the program locally using WebStart.
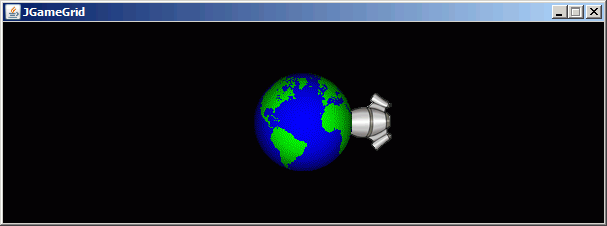
| |