|
The source code of all examples is included in the JGameGrid distribution.
Ex15: Check Button Actors
Check buttons are used to make a non-exclusive selection of a boolean value, e.g. to answer a yes-no question. They are common for setting-up application initial conditions. The GGCheckButton class is a special type of a button, therefore derived from GGButtonBase that subclasses Actor. The button appearance is a square of given size for the unchecked state with a diagonal cross for the checked state. An annotation text is placed to the right of the square. This is a somewhat restricted choice, but smarter check buttons are easily implemented using GGToogleButton.
The corresponding actor of a check button is actually a rectangle with the height of the square and the length roughly twice the text length plus the square size. The square is positioned in the middle of the rectangle. The left side of the rectangle is transparent and the right side contains the text. Because the center of the actor's area is at the center of the square, several check buttons may be easily vertically aligned. For fine positioning the buttons in a GameGrid windows with a coarse grid, the location may be moved by a fixed pixel offset by using GameGrid.setLocationOffset().
Changes from the unchecked to the checked state and vice versa are reported by an event callback buttonChecked() defined in the GGCheckButtonListener interface. As with the GGButton class, events are inserted into a Fifo queue and the callback method is invoked by the separate button event thread.
In the following example there are two check buttons to run/pause the program and to modify the simulation period. The default text and background colors of GGCheckButton are used, because the playground's background is white. The speed check button is initially checked by setting the boolean constructor parameter.
import ch.aplu.jgamegrid.*;
import java.awt.*;
public class Ex15 extends GameGrid implements GGCheckButtonListener
{
private Actor car = new Actor(true, "sprites/car.gif");
private GGCheckButton cb1 = new GGCheckButton("Run");
private GGCheckButton cb2 = new GGCheckButton("Fast", true);
public Ex15()
{
super(300, 300, 1, false);
setSimulationPeriod(50);
setBgColor(Color.white);
addActor(car, new Location(150, 50));
addActor(cb1, new Location(150, 140));
cb1.addCheckButtonListener(this);
addActor(cb2, new Location(150, 160));
cb2.addCheckButtonListener(this);
show();
}
public void act()
{
car.move();
car.turn(2.5);
}
public void buttonChecked(GGCheckButton button, boolean checked)
{
if (button == cb1)
{
if (checked)
doRun();
else
doPause();
}
if (button == cb2)
{
if (checked)
setSimulationPeriod(50);
else
setSimulationPeriod(100);
}
}
public static void main(String[] args)
{
new Ex15();
}
}
|
Execute the program locally using WebStart.
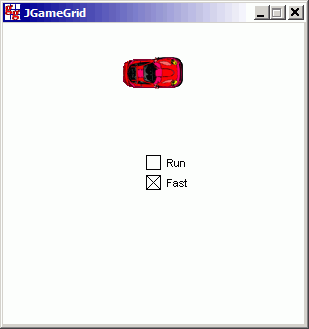 |
|
|
Check Buttons Ex15 |
|
Radio Buttons Ex16 |
Ex16: Radio Button Actors
Normally radio buttons are used for an exclusive selection of a certain number of boolean values, e.g. to select one of several proposals. To achieve this, the radio buttons are grouped together in a GGRadioButtonGroup that is responsible to allow one and only one button to be selected. The GGRadioButton class is a special type of a GGButton, thus derived from GGButtonBase that subclasses Actor. The button appearance is a circle of given size for the deselected state with a center spot for the checked state. An annotation text is placed to the right of the circle. Like for GGCheckButton this is a somewhat restricted choice, but smart radio buttons are easily implemented using GGToogleButton.
The layout of the corresponding actor is the same as for check buttons. Ex15 gives more information if needed.
Changes from the deselected to the selected state and vice versa are reported by an event callback buttonSelected() defined in the GGRadioButtonListener interface. As with the GGButton class, events are inserted in a Fifo queue and the callback method is invoked by a separate button event thread. Adding a radio button into more than one button radio group is not allowed.
In the following example the sprite image of a car actor is changed at run-time by clicking one of the three radio buttons. Because the GGRadioButtonListener is registered in the GGRadioButtonGroup, only 'select' events are reported, so the parameter selected is always true. The GGRadioButtonGroup constructor accepts any number of GGRadioButton references because it uses ellipsis (variable length argument list). To insert a button into a button group that is already constructed, GGRadioButtonGroup.add() may be used that also supports ellipsis.
import ch.aplu.jgamegrid.*;
import java.awt.*;
public class Ex16 extends GameGrid implements GGRadioButtonListener
{
private Actor car = new Actor(true, "sprites/car.gif", 3);
private GGRadioButton redBtn = new GGRadioButton("Red", true);
private GGRadioButton greenBtn = new GGRadioButton("Green");
private GGRadioButton blueBtn = new GGRadioButton("Blue");
public Ex16()
{
super(300, 300, 1, false);
setSimulationPeriod(50);
setBgColor(Color.white);
addActor(car, new Location(150, 50));
addActor(redBtn, new Location(150, 140));
addActor(greenBtn, new Location(150, 160));
addActor(blueBtn, new Location(150, 180));
GGRadioButtonGroup group =
new GGRadioButtonGroup(redBtn, greenBtn, blueBtn);
group.addRadioButtonListener(this);
show();
doRun();
}
public void act()
{
car.move();
car.turn(2.5);
}
public void buttonSelected(GGRadioButton button, boolean checked)
{
if (button == redBtn)
car.show(0);
if (button == greenBtn)
car.show(1);
if (button == blueBtn)
car.show(2);
}
public static void main(String[] args)
{
new Ex16();
}
} |
Execute the program locally using WebStart.
| |