|
The source code of all applications is included in the JGameGrid distribution.
LightsOut
The game consists of a 5 by 5 grid of lights; when the game starts, a set of these lights (random, or one of a set of stored puzzle patterns) are switched on. Pressing one of the lights will toggle it and the four lights adjacent to it on and off. (Diagonal neighbours are not affected.) The game provides a puzzle: to switch all the lights off, preferably in as few button presses as possible.
Lights Out was invented by a group of people consisting of Avi Olti, Gyora Benedek, Zvi Herman, Revital Bloomberg, Avi Weiner and Michael Ganor.
The game involves toggling lights on and off. If a light is on, it must be toggled an odd number of times to be turned off. If a light is off, it must be toggled an even number of times (including not being toggled at all) for it to remain off. A successful solution is therefore a sequence of presses that toggles all the "on" lights an odd number of times and all the "off" lights an even number of times.
Two points may be noted:
- The order in which the lights are pressed does not matter. The end result of pressing a given set of lights is always that each light has been toggled a certain number of times
- Each light needs to be pressed no more than once. Note that pressing a light twice is equivalent to not pressing it at all. Since the order in which the lights are pressed does not matter, a sequence in which one light is pressed twice is equivalent to the same sequence with those two presses removed. Hence, the most efficient method of solving any puzzle (one that uses the minimum number of moves) is one in which no light is pressed more than once
Despite these results, in practice, given a random board setup, it will not be obvious what sequence will toggle the "on" and "off" lights the required number of times.
[Ref. http://en.wikipedia.org/wiki/Lights_Out_(game)]
This example demonstrates that developing a full-fledged game that achieves professional standards with the JGameGrid framework reduces the programming effort an order of magnitude. Only rudimental knowledge of Java and a few lines of code are necessary to implement the game in its basic but already playable version. It is proven to be a problem that can be solved in an entry level programming course. We present here one of many possible solutions where an ArrayList is used (instead of considering the 8 neighbour cells explicitly). The two states of the lamps (off/on) are shown by using an actor with two sprite ids.
A GGMouseListener is registered to get the left press mouse event exclusively. Because the neighbour locations may be outside the grid (where getOneActorAt() returns null), isInGrid() is called to test for valid grid locations. The convenient method showNextSprite() runs through all sprite ids. Here it just toggles between id 0 and 1.
Aimport ch.aplu.jgamegrid.*;
import java.util.*;
import java.awt.*;
public class LightsOutLight extends GameGrid implements GGMouseListener
{
public LightsOutLight()
{
super(5, 5, 50, Color.black, false);
setTitle("LightsOutLight");
for (int i = 0; i < 5; i++)
{
for (int k = 0; k < 5; k++)
{
Actor actor = new Actor("sprites/lightout.gif", 2);
addActor(actor, new Location(i, k));
actor.show(1);
}
}
addMouseListener(this, GGMouse.lPress);
show();
}
public boolean mouseEvent(GGMouse mouse)
{
Location location = toLocationInGrid(mouse.getX(), mouse.getY());
ArrayList<Location> locations = location.getNeighbourLocations(0.5);
locations.add(new Location(location.x, location.y));
for (Location loc : locations)
{
if (isInGrid(loc))
{
Actor a = getOneActorAt(loc);
a.showNextSprite();
}
}
refresh();
return true;
}
public static void main(String[] args)
{
new LightsOutLight();
}
} |
Execute the program locally using WebStart.
The basic version may be extended step-by-step that gives enough challange for more advanced students. You may even include a solution finder like in the following program (the solution algorithm was presented by David Guichard, Whitman College, USA).
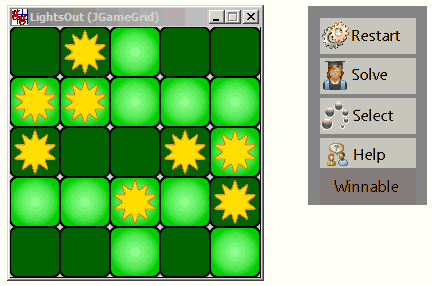
Execute the program locally using WebStart
| |